File
Description
Manages general settings for Config page
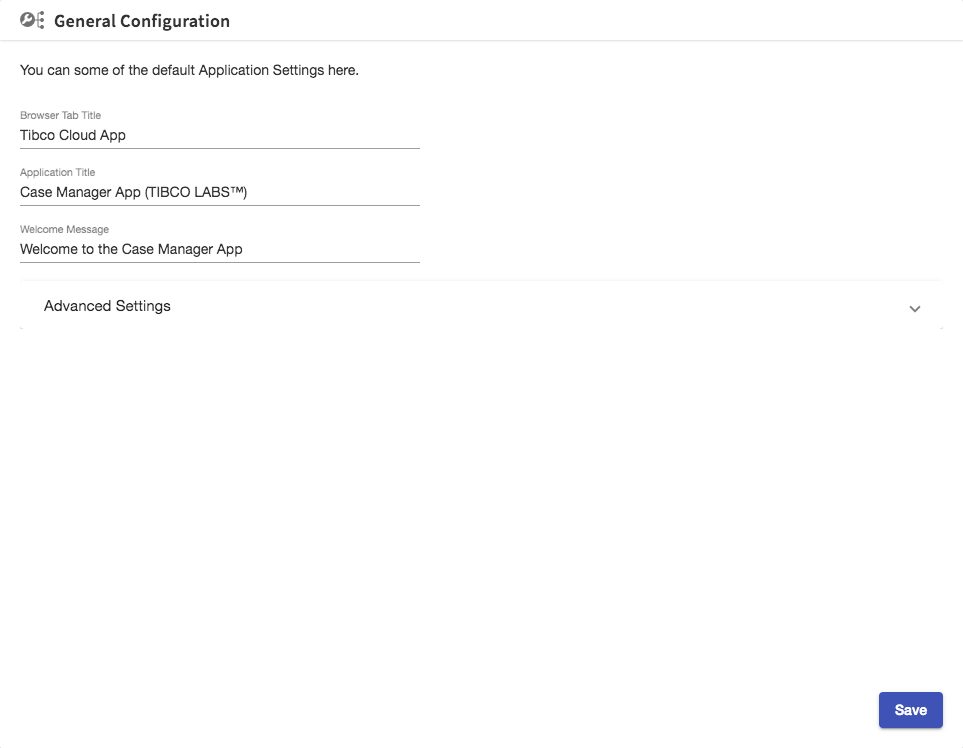
Implements
Example
<tc-tibco-cloud-settings-general></tc-tibco-cloud-settings-general>
Metadata
selector |
tc-tibco-cloud-settings-general |
styleUrls |
./tibco-cloud-settings-general.component.css |
templateUrl |
./tibco-cloud-settings-general.component.html |
Methods
Protected
getRoute
|
getRoute()
|
|
|
Public
applicationTitle
|
Type : string
|
|
Public
claims
|
Type : Claim
|
|
Public
documentationURL
|
Type : string
|
|
Public
panelOpenState
|
Default value : false
|
|
Public
runSaveFunction
|
Default value : () => {...}
|
|
Public
sandboxId
|
Type : number
|
|
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { GeneralConfig } from '../../models/tc-general-config';
import { TcGeneralConfigService } from '../../services/tc-general-config.service';
import { Claim } from '../../models/tc-login';
import { MatSnackBar } from '@angular/material/snack-bar';
/**
* Manages general settings for Config page
*
* 
*
*@example <tc-tibco-cloud-settings-general></tc-tibco-cloud-settings-general>
*/
@Component({
selector: 'tc-tibco-cloud-settings-general',
templateUrl: './tibco-cloud-settings-general.component.html',
styleUrls: ['./tibco-cloud-settings-general.component.css']
})
export class TibcoCloudSettingsGeneralComponent implements OnInit {
public applicationTitle: string;
public roles;
public displayName: boolean;
public documentationURL: string;
public panelOpenState = false;
public generalConfig: GeneralConfig;
public sandboxId: number;
public claims: Claim;
constructor(protected route: ActivatedRoute, protected generalConfigService: TcGeneralConfigService, protected snackBar: MatSnackBar) { }
ngOnInit() {
this.generalConfig = this.route.snapshot.data.generalConfigHolder;
this.claims = this.route.snapshot.data.claims;
this.sandboxId = Number(this.claims.primaryProductionSandbox.id).valueOf();
this.applicationTitle = this.generalConfig.applicationTitle;
// this.roles = this.generalConfig.roles;
this.displayName = this.generalConfig.displayName;
this.documentationURL = this.generalConfig.documentationUrl;
}
protected getRoute(): ActivatedRoute {
return this.route;
}
public runSaveFunction = () => {
this.generalConfigService.updateGeneralConfig(this.sandboxId, this.generalConfig.uiAppId, this.generalConfig, this.generalConfig.id).subscribe(
result => {
this.snackBar.open('General configuration saved', 'OK', {
duration: 3000
});
},
error => {
this.snackBar.open('Error saving general configuration saved', 'OK', {
duration: 3000
});
}
);
}
}
<div fxLayout="column" fxFill>
<tc-tibco-cloud-widget-header style="height: 40px;" [icon]="'tcs-capabilities'"
[headerText]="'General Configuration'"></tc-tibco-cloud-widget-header>
<div fxFlex style="padding: 20px; overflow: hidden" fxLayout="column">
<div style="overflow: auto;">
<div fxLayout="column" fxFlex>
<p>You can some of the default Application Settings here.</p>
<br>
<!-- Application specifics -->
<div class="tcs-app-form-field" fxLayout="row">
<mat-form-field style="min-width: 400px">
<input matInput placeholder="Browser Tab Title" [(ngModel)]="generalConfig.browserTitle">
</mat-form-field>
</div>
<div class="tcs-app-form-field" fxLayout="row">
<mat-form-field style="min-width: 400px">
<input matInput placeholder="Application Title" [(ngModel)]="generalConfig.applicationTitle">
</mat-form-field>
</div>
<div class="tcs-app-form-field" fxLayout="row">
<mat-form-field style="min-width: 400px">
<input matInput placeholder="Welcome Message" [(ngModel)]="generalConfig.welcomeMessage">
</mat-form-field>
</div>
<!-- Advanced Settings (all Optional from Shared State) -->
<mat-accordion>
<mat-expansion-panel>
<mat-expansion-panel-header>
<mat-panel-title>Advanced Settings</mat-panel-title>
</mat-expansion-panel-header>
<mat-form-field style="min-width: 400px">
<input matInput placeholder="Application Documentation URL" [(ngModel)]="generalConfig.documentationUrl">
</mat-form-field>
</mat-expansion-panel>
</mat-accordion>
</div>
</div>
<div fxFlex class="tcs-filler-panel"></div>
<div fxLayout="column" fxLayoutAlign="end end" style="min-height: 50px">
<button mat-raised-button color="primary" (click)="runSaveFunction()">Save</button>
</div>
</div>
</div>
.tcs-app-form-field {
min-height: 57px;
}
Legend
Html element with directive