File
Description
This component will attempt to log the user in.
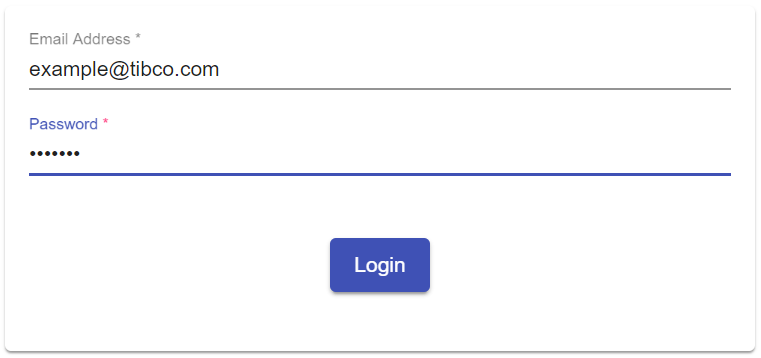
Implements
Example
<tc-tibco-cloud-login *ngIf="!loggedIn (loggedIn)="handleLoggedIn($event)"></tc-tibco-cloud-login>
Metadata
selector |
tc-tibco-cloud-login |
styleUrls |
./tibco-cloud-login.component.css |
templateUrl |
./tibco-cloud-login.component.html |
Index
Properties
|
|
Methods
|
|
Inputs
|
|
Outputs
|
|
Accessors
|
|
Constructor
constructor(tcLogin: TcLoginService)
|
|
The Constructor creates the Login Dialog
|
Outputs
loggedIn
|
Type : EventEmitter
|
|
Notify parent that user is logged in ok.
|
Methods
handleSignUp
|
handleSignUp()
|
|
|
handleUseOauth
|
handleUseOauth()
|
|
|
Public
appName
|
Type : string
|
Default value : 'Cloud Starters'
|
|
|
loading
|
Default value : false
|
|
matExpansionPanel
|
Type : MatExpansionPanel
|
Decorators :
@ViewChild(MatExpansionPanel, {static: false})
|
|
Accessors
AppName
|
setAppName(appName: string)
|
|
Parameters :
Name |
Type |
Optional |
appName |
string
|
No
|
|
import {Component, EventEmitter, Input, OnChanges, OnInit, Output, SimpleChanges, ViewChild} from '@angular/core';
import {AccountsInfo, LoginPrefill, Subscription} from '../../models/tc-login';
import {Observable, ObservableInput} from 'rxjs';
import {map, mergeMap} from 'rxjs/operators';
import {AccessToken, AuthInfo} from '../../models/tc-login';
import {TcLoginService} from '../../services/tc-login.service';
import {MatExpansionPanel} from '@angular/material/expansion';
/**
* This component will attempt to log the user in.
*
* 
*
* @example <tc-tibco-cloud-login *ngIf="!loggedIn (loggedIn)="handleLoggedIn($event)"></tc-tibco-cloud-login>
*/
@Component({
selector: 'tc-tibco-cloud-login',
templateUrl: './tibco-cloud-login.component.html',
styleUrls: ['./tibco-cloud-login.component.css']
})
export class TibcoCloudLoginComponent implements OnChanges {
/**
* Notify parent that user is logged in ok.
*/
@Output() loggedIn = new EventEmitter();
/**
* Output useOauth event
*/
@Output() useOauth = new EventEmitter();
/**
* Output signUp event
*/
@Output() signUp = new EventEmitter();
/**
* Login Data
*/
@Input() loginPrefill: LoginPrefill;
/**
* App Name
*/
public appName = 'Cloud Starters';
@Input('appName') set AppName(appName: string) {
if (appName) {
this.appName = appName;
}
}
@ViewChild(MatExpansionPanel, { static: false }) matExpansionPanel: MatExpansionPanel;
name: string;
password: string;
clientId: string;
loading = false;
accountsInfo: AccountsInfo;
error: string;
token: AccessToken;
authInfo: AuthInfo;
auth: Observable<AuthInfo>;
/**
* The Constructor creates the Login Dialog
*/
constructor(
private tcLogin: TcLoginService
) {
}
doLogin() {
if (this.matExpansionPanel && this.matExpansionPanel.expanded) {
this.matExpansionPanel.expanded = false;
}
this.loading = true;
this.error = undefined;
this.auth = this.tcLogin.login(this.name, this.password, this.clientId).pipe(
map((authInfo: AuthInfo) => {
this.authInfo = authInfo;
return authInfo;
}
)
);
this.auth.subscribe(authorize => {
this.loading = false;
// ok logged in
console.log('User logged in...');
// update claims
this.loggedIn.emit({authInfo: authorize, accessToken: this.token});
},
error => {
this.loading = false;
if (error.error && error.error.errorMsg) {
this.error = error.error.errorMsg;
}
console.error('Login Failed: ');
console.error(error);
});
}
handleUseOauth() {
this.useOauth.emit();
}
handleSignUp() {
this.signUp.emit();
}
ngOnChanges(changes: SimpleChanges): void {
if (this.loginPrefill) {
this.name = this.loginPrefill.emailId;
this.clientId = this.loginPrefill.clientId;
}
}
}
<div class="login-container">
<div class="login-screen">
<div class="login-container-box">
<div class="login-box">
<div class="login-inner-box">
<div class="welcome-text">Welcome to</div>
<div class="welcome-content">
<mat-icon svgIcon="ic-tibco-logo" class="tibco-logo"></mat-icon>
<div class="app-text">{{appName}}</div>
</div>
<div class="login-container-form">
<form class="login-fields" #laLoginForm="ngForm" class="la-login-form" (submit)="doLogin()" fxLayout="column" fxFlex disabled="loading">
<mat-form-field>
<input matInput type="text" placeholder="Email Address" [(ngModel)]="name" name="email" required>
</mat-form-field>
<mat-form-field>
<input matInput type="password" placeholder="Password" [(ngModel)]="password" name="password" required>
</mat-form-field>
<mat-accordion>
<mat-expansion-panel [expanded]="!clientId">
<mat-expansion-panel-header>
<mat-panel-title>API Access Keys</mat-panel-title>
</mat-expansion-panel-header>
<div fxLayout="column">
<mat-form-field fxFlex>
<input matInput placeholder="Client Id" [(ngModel)]="clientId" name="ClientID" required>
</mat-form-field>
</div>
</mat-expansion-panel>
</mat-accordion>
<div class="sign-in-section">
<button style="width: 144px;" type="submit" mat-raised-button color="primary" type="submit" [disabled]="(!laLoginForm.form.valid || !clientId) || loading">Login</button>
</div>
</form>
</div>
<mat-spinner *ngIf="loading" class="spinner" diameter="40"></mat-spinner>
<div *ngIf="error" class="error-text">{{error}}</div>
<div class="sign-up-section">
<div class="sign-up-text">
<span>Have an oauth key? </span>
<a (click)="handleUseOauth()">Use oauth authentication</a>
<br>
<span>Don’t have an account? </span><a (click)="handleSignUp()">Sign up</a>
</div>
</div>
</div>
</div>
</div>
<div class="copyright-text">
<span>Copyright © 2021 TIBCO Software Inc. All Rights Reserved.</span>
</div>
</div>
</div>
.la-login-card {
}
.la-login-form {
}
.la-login-div {
min-width: 300px;
}
.la-login-button {
width: 50px;
}
.la-login-info-pane {
min-height: 50px;
height: 50px;
max-height: 50px;
margin-top: 10px;
}
.spacer {
min-height: 0px;
}
.la-login-button-div {
margin-top: 25px;
}
.login-container-box {
flex: 1;
align-items: center;
display: flex;
}
.login-container {
height: 100%;
display: flex;
flex-direction: column;
}
.login-screen {
height: 100%;
width: 100%;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
.login-box {
height: 472px;
width: 424px;
border-radius: 10px;
background-color: #FFFFFF;
box-shadow: 0 2px 8px 0 rgba(221,221,221,0.88);
}
.login-inner-box {
padding: 32px;
height: 100%;
display: flex;
flex-direction: column;
overflow: hidden;
}
.welcome-text {
color: #1774E5;
font-family: var(--app-font-extra-light, Source Sans Pro);
font-size: 18px;
letter-spacing: 0;
line-height: 21px;
align-self: center;
display: flex;
justify-content: center;
}
.welcome-content {
display: flex;
flex-direction: row;
justify-content: center;
padding-left: 10px;
padding-right: 10px;
margin-top: 8px;
align-items: center;
}
.spinner {
display: flex;
flex-direction: row;
align-self: center;
margin-top: 15px;
}
.tibco-logo {
margin-bottom: 3px;
display: flex;
flex-direction: row;
align-self: center;
width: 123px;
height: 32px;
}
.app-text {
color: #1774E5;
font-family: var(--app-font-extra-light, Source Sans Pro);
font-size: 36px;
letter-spacing: -1px;
line-height: 38px;
}
.login-fields {
margin-top: 20px;
width: 100%;
}
.login-fields > mat-form-field {
width: 100%;
}
.sign-in-section {
margin-top: 30px;
display: flex;
flex-direction: row;
justify-content: center;
}
.sign-up-section {
display: flex;
flex-direction: row;
flex: 1;
align-items: flex-end;
}
.sign-up-text {
display: inline-block;
width: 350px;
color: #212121;
font-family: var(--app-font-extra-light, Source Sans Pro);
font-size: 12px;
letter-spacing: 0;
line-height: 18px;
text-align: center;
}
.sign-up-text a {
color: #1774E5;
font-family: var(--app-font-extra-light, Source Sans Pro);
font-size: 12px;
letter-spacing: 0;
line-height: 18px;
text-align: center;
}
.error-text {
display: flex;
color: red;
font-family: var(--app-font-extra-light, Source Sans Pro);
font-size: 12px;
font-weight: 600;
letter-spacing: 0;
line-height: 27px;
justify-content: center;
}
.copyright-text {
display: flex;
color: #4A4A4A;
font-family: "Source Sans Pro";
font-size: 13px;
letter-spacing: 0;
line-height: 20px;
text-align: center;
align-items: flex-end;
margin-bottom: 20px;
}
.login-container-form {
margin-top: 20px;
}
a:hover {
cursor: pointer;
}
Legend
Html element with directive